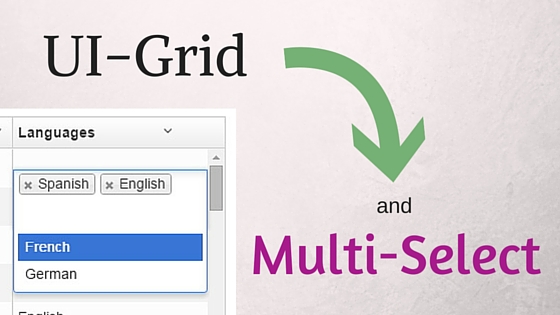
In a previous post we explored how to use dropdown widgets with UI-Grid. That’s great if your model allows just one value, but what if you want to allow multiple values?
Here’s an example: you’ve got a list of people who can each speak one or more languages. Your users need to be able select multiple options from a list of languages for each person in your data set. How do you do it?
Thankfully, UI-Select already has multi-select built in. All we need to do is take the code from our previous post and tweak it a bit.
Tweak the Theme
First off, it doesn’t look like we can use the selectize theme for multi-select.
We’ll have to use select2. This is as simple as swapping out the theme property
in our ui-select directive: theme="select2"
.
That only changes the theme; it doesn’t enable multi-selection. In order to do that we need to
also add the multiple
property.
We’ll also need to swap out our reference to the selectize CSS for select2’s:
https://cdnjs.cloudflare.com/ajax/libs/select2/3.4.5/select2.min.css
This will have our select box looking like this:
Comma Separated Values
Next, in our grid we want to see the values separated by commas. We can create a custom cell template
that joins the column’s field with the join
operator:
1
2
3
|
<div
class="ui-grid-cell-contents">
{{ COL_FIELD.join(', ') }}
</div>
|
There’s other ways you could choose to display the selected values, of course. Comma-separated is easy to implement though.
Change the Placeholder
Finally, we need to make sure the selected options show up properly. Inside the
ui-select-match
directive we change {{ blah }}
to {{ $item }}
.
Our final UI-Select markup looks like this:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
<ui-select-wrap>
<ui-select
multiple
ng-model="MODEL_COL_FIELD"
theme="select2"
ng-disabled="disabled"
append-to-body="true">
<ui-select-match placeholder="Choose...">{{ $item }}</ui-select-match>
<ui-select-choices repeat="item in
col.colDef.editDropdownOptionsArray | filter: $select.search">
<span>{{ item }}</span>
</ui-select-choices>
</ui-select>
</ui-select-wrap>
|
Example
Here’s our updated plunker:
Have questions or suggestions? Leave them in the comments below!